
THIS MAGENTO HACK IS NOW A MAGENTO MODULE/EXTENSION, CLICK HERE FOR MORE INFO
Wouldn’t it be great if your customers could do your twitter product marketing for you? Thats what I thought after seeing a few automated marketing posts coming up in my twitter feed. So I set about looking at my own automated marketing tweets triggered when a customer placed an order in our Magento e-commerce store. The goal was to randomly select a product from the last order – a so called “Top Seller”, wrap it up nicely in a 140 char tweet with a URL to the product page and post it to twitter automatically from the onepage checkout success page as the customer completed checkout. After a day or so of development I came up with a solution that looks something like this on Magento 1.3.3.0 using the test database.

Step 1 – Installing in Magento
To integrate the code into Magento you can either use the onepage checkout success.phtml file to call the code after checkout, or if you have already developed your own Magento code modules you can create a new template phtml file and test the code by calling it from a CMS block in Magento i.e.
<div>{{block type="MY_Page/view" template="Page/tweet.phtml"}}</div>
If you use success.phtml you will need to submit an order from your webshop to test the code, if you are using CMS simply browse to the CMS page you created, i.e. http://www.mysite.com/tweet
Here is the working code to use either in your own phtml template file or to append to success.phtml in your frontend themes template files.
<?php // Magento Product Twitter Marketing // // paj // www.gaiterjones.com // tested with Magento 1.3.3.0 // v1.0 // Short URL function function make_bitly_url($url,$login,$appkey,$format = 'xml',$history = 1) { //create the URL $bitly = 'http://api.bit.ly/v3/shorten?login='.$login.'&apiKey='.$appkey.'&uri='.urlencode($url). '&format='.$format.'&history='.$history; //get the url $response = file_get_contents($bitly); //parse depending on desired format if(strtolower($format) == 'json') { $json = @json_decode($response,true); return $json['data']['url']; } elseif(strtolower($format) == 'xml') //xml { $xml = simplexml_load_string($response); return $xml->data->url; } elseif(strtolower($format) == 'txt') //text { return $response; } } // Get last order from Magento session. $orderId = Mage::getModel('sales/order')->load(Mage::getSingleton('checkout/session')->getLastOrderId()); $order = Mage::getModel('sales/order')->load($orderId); $items = $order->getAllItems(); $itemcount=count($items); //$name=array(); //$unitPrice=array(); //$sku=array(); $ids=array(); //$qty=array(); try { // Build arrays, uncomment as needed to populate arrays with more order information, SKU, price etc. foreach ($items as $itemId => $item) { // $name[] = $item->getName(); // $unitPrice[]=$item->getPrice(); // $sku[]=$item->getSku(); // we are only interested in the product id $ids[]=$item->getProductId(); // $qty[]=$item->getQtyToInvoice(); // echo $name[$itemId]. ' - ' .$sku[$itemId]. ' - €'. $unitPrice[$itemId]. '<br>'; } do { shuffle ($ids); $rand_keys = (count($ids) > 1)?array_rand($ids, $itemcount):array_keys($ids); $custom = Mage::getModel('catalog/product')->load($ids[$rand_keys[0]]); // Make sure the product is visible in catalog if ($custom->getVisibility()==4) { // twitter text prefix, this will appear before your product description $twitter_txt=$this->__('Top Seller'). ": "; // twitter txt URL - add a short URL to the product using bit.ly API $shorturl=make_bitly_url($custom->getProductUrl(),'YOUR-BITLY-USERNAME','YOUR-BITLY-API'); // The text between our tweet and the shorturl when the tweet is longer than 140 chars $twitter_txt_suffix = '... '; $chars_remaining=140-(strlen($shorturl)+strlen($twitter_txt_suffix)); // debugging echos comment these out when testing complete. // Check lengths of short url, prefix etc. echo $shorturl. ' is '. strlen($shorturl). ' chars.<br>'; echo 'Suffix and URL are '. (strlen($shorturl)+strlen($twitter_txt_suffix)). ' chars.<br>'; echo 'Characters remaining for tweet : '. $chars_remaining. '<br>'; // Prepare tweet text $twitter_txt=$twitter_txt . strip_tags(str_replace("<br />",", ",substr($custom->getDescription(), 0, strpos($custom->getDescription(), '.')+1))). ' '; // Make sure we only tweet 140 chars or less if (strlen($twitter_txt) <= $chars_remaining) { $twitter_txt = $twitter_txt; //do nothing } else { $twitter_txt = wordwrap($twitter_txt, $chars_remaining); $twitter_txt = substr($twitter_txt, 0, strpos($twitter_txt, "\n")); $twitter_txt = $twitter_txt. $twitter_txt_suffix; } // Complete tweet text with short url. $twitter_txt = $twitter_txt. ' ' .$shorturl; // Get ready to tweet $accessToken = file_get_contents("/home/www/classes/twitteroauth/access_token"); $accessTokenSecret = file_get_contents("/home/www/classes/twitteroauth/access_token_secret"); // Create our twitter API object require_once('/home/www/classes/twitteroauth/twitteroauth.php'); $oauth = new TwitterOAuth('YOUR-TWITTER-CONSUMER-KEY', 'YOUR-TWITTER-CONSUMER-SECRET', $accessToken, $accessTokenSecret); // Send an API request to verify credentials $credentials = $oauth->get("account/verify_credentials"); echo "Connected as @" . $credentials->screen_name. '<br>'; // TWEEET, uncomment to tweet. //$oauth->post('statuses/update', array('status' => $twitter_txt)); // debugging echos comment these out when testing complete. echo 'Simulated tweet : ('. strlen($twitter_txt). ' chars long.)<br><br>'; echo '<strong>'. $twitter_txt. '</strong><br>'; } } while ($custom->getVisibility()!=4); // debugging use this to catch any errors, remove error message when testing complete. } catch (Exception $ex) { echo "An Error was detected."; } ?>
Copy and paste the code at the end of your one page checkout success.phtml file or paste it into a new template phtml file i.e. tweet.phtml.
The first function is used to create a shortened URL via the bit.ly API, for this you will need a bit.ly account and your bit.ly API key (available form the settings menu in your account). We are only really interested in the product ids from the order, but if you want to use other information in your tweet remove the comments from the array loop.
$twitter_txt contains our tweet text. It starts out with a prefix “Top Sellers” and then uses an excerpt from the Magento product description to create the rest of the tweet text.
$twitter_txt=$twitter_txt . strip_tags(str_replace("<br />",", ",substr($custom->getDescription(),0, strpos($custom->getDescription(), '.')+1))). ' ';
My product descriptions text always includes the product name on the first line of the long description text followed by a line break e.g.
<p><strong>Nokia 2610 Phone</strong><br />The Nokia 2610 is an easy to use device that combines multiple messaging options including email, instant messaging, and more.
We strip out the HTML replacing the first html line break with a comma and then search for the next full stop to extract the first sentence from our description. (This is also a good technique I have used for automatic SEO meta description tags for products.) If your product descriptions do not include line breaks you will need to play with this code so that it extracts a product description that suits your requirements.
We need to make sure our tweet including prefix and short url are less than 140 chars so we use some code to check how long the tweet product description text is and shorten it if required adding a “…” suffix. Now we have our tweet text and call the Twitter oAuth code to authenticate and post the tweet. The code that makes the tweet is commented, and for testing the tweet text will be echoed out so you can easily debug your tweet and description text, the simulated tweet should look something like this:
http://bit.ly/hd6Et1 is 20 chars. Suffix and URL are 24 chars. Characters remaining for tweet : 116 Connected as @your-twitter-username Simulated tweet : (135 chars long.) Top Seller: Nokia 2610 Phone, The Nokia 2610 is an easy to use device that combines multiple messaging options... http://bit.ly/hC6Et1
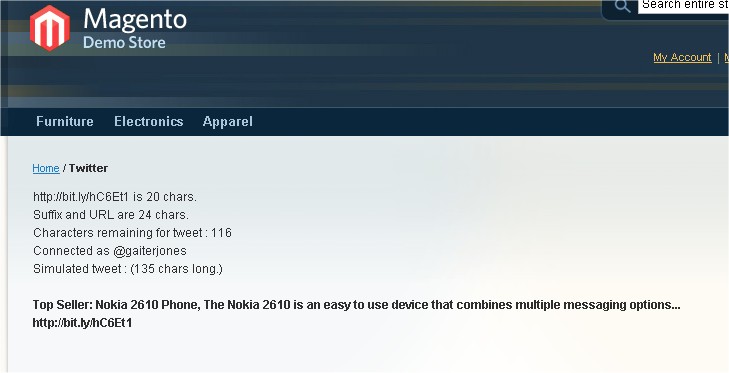
See below for setting up your twitter application and installing the oAuth code.
Note that you need to have an order in the current magento session, I haven’t yet figured out how to access the last order outwith the user session, so if you don’t see anything or see the exception error echoed out make sure you are logged into your magento shop and have placed a test order.
Step 2 – Twitter PHP oAuth Code
The Twitter oAuth authentication process is complex and way beyond my programming skills, however Abraham Williams has developed the PHP code to perform the twitter oAuth authentication and posting for us. Download the code here. Open the ZIP folder and extract the twitteroauth folder to a suitable area on your development host. For example /home/www/classes. The folder contains two PHP files, twitteroauth.php and OAuth.php that are called from our code, update the script include so that the path to your oAuth files is correct.
Step 3 – Twitter Application and Access Codes
Obviously to create a twitter app you need a twitter account. Login to your twitter account and go to http://dev.twitter.com/apps/new to register a new twitter app. Complete the registration by choosing an application name, note this name will appear under your posts! Set the application type to client and make sure you set the application type to read & write.
When you have registered your application take a note of the Consumer Key and Consumer Secret. Click on the My Access Token right hand menu link and take a note of your Access Token (oauth_token) and Access Token Secret (oauth_token_secret).
Copy and paste your Twitter Consumer Key and Consumer Secret into the script.
$oauth = new TwitterOAuth('YOUR-TWITTER-CONSUMER-KEY','YOUR-TWITTER-CONSUMER-SECRET', $accessToken, $accessTokenSecret);
The Twitter Access Token and Access Token Secret can be included in plain text in the script but for security I am separating them out into two seperate files located with my oauth PHP scripts:
$accessToken = file_get_contents("/home/www/classes/twitteroauth/access_token"); $accessTokenSecret = file_get_contents("/home/www/classes/twitteroauth/access_token_secret");
So either modify the strings above to contain your access codes as plain txt i.e. $accessToken=”MY-ACCESS-TOKEN”; or create your own folder and paths to two files containing your twitter access keys in plain text.
Step 4 – Test
If you have copied the code to your success.phtml file make an order and you should see the simulated tweet with your product description on your one page checkout success page.
http://bit.ly/hd6Et1 is 20 chars. Suffix and URL are 24 chars. Characters remaining for tweet : 116 Connected as @your-twitter-username Simulated tweet : (135 chars long.) Top Seller: Nokia 2610 Phone, The Nokia 2610 is an easy to use device that combines multiple messaging options... http://bit.ly/hC6Et1
To make a test tweet uncomment the tweet statement and place another order, check your twitter account for a new tweet!
When you are happy with your tweet text, uncomment the debugging echos from the script and test again.

Conclusions and further ideas
Twitter marketing can easily become Twitter spam, depending on how many orders a day your shop is getting you may need to throttle back the number of product tweets you make, the tweet could also be handled elsewhere in the app, or from a cron job that picks a random product each day. I like the idea of the “Top Seller” from an actual order, and that the customer was actually doing the marketing work for you! You may also want to extract your parent categories to create additional hash tags for your tweets to improve search results, although this will eat up your precious 140 chars.
Some Googled resources used in creating this solution:
http://articles.sitepoint.com/article/oauth-for-php-twitter-apps-part-1
http://subesh.com.np/2010/03/ordered-items-detail-order-id-magento/
Very Good Article.
Thanks
BharatMatrimony
This is great!
Where in the code could I add a tracking code to the URL so it would be included in the shorting process?
You could add a query string to the URL when you create the shorturl using something like :
$shorturl=make_bitly_url($custom->getProductUrl(). '&trackingcode=12345','YOUR-BITLY-USERNAME','YOUR-BITLY-API');
Thanks PAJ!
I was able to successfully add the tracking code to the bit.ly url. However, I’m having an issue getting the actual tweet to work though. In testing, I get this on success.phtml after checking out:
http://bit.ly/oCgrZc is 20 chars.
Suffix and URL are 24 chars.
Characters remaining for tweet : 116
But there is no indication of Twitter connection or sample tweet information.
I’ve created my Twitter app, entered all tokens and consumer key info, uploaded the OAuth files, updated the script to point to the correct files… but still no luck on the tweet.
Any insights greatly appreciated!
Hi Chris,
Been a while since I developed this but I have used the tweet method a lot in various apps without any problems. It does sound like it is just the tweet process that is failing which is likely to be a problem with your twitter account, double check everything is set up ok with your twitter app. If your dev server is accessible would be happy to have a look for you. Have been wanting to adapt this into a module for Magento for ages to make it easier to use…
PAJ,
I’m going to triple check all the app setting, tokens, keys, etc. this morning. I’ll be more than happy to give access to my server for you to check all my work. you can email me – chris.hood at shubee dot com.
Thanks again for all your help and support, really looking forward to getting this project complete!
Hi,
Is this able to work with new products? ie, when a new product is added, it will auto tweet? I can obviously pay for this development. can you contact me please?
thanks